Problem-Solving Skills for the Programming Interview
Draft of the course proposed for Spring 2019
Spring 2019: CCOM4995-043
- Description:
- Solving real-world computational problems requires a good working knowledge of data structures and algorithms. Questions that appear in programming interviews can have deceivingly simple statements. However the first solution is almost never the best. Thinking through the obstacles in order to obtain a better solution is a discovery process that leads to a deeper understanding about what certain algorithms and data structures do, and why people studied them in the first place. This course aims to help the student be more prepared for a programming interview by acquiring a working knowledge of data structures and algorithms through programming interview questions, along with a dialectical in-class demonstration of problem-solving methodologies. (Taken from Online Reference [1] )
Syllabus: [PDF]
- Prerequisites:
- CCOM3034 Data Structures
Objectives:
At the end of this course the student will be able to:
- Describe problem-solving strategies/approaches, e.g. IDEAL, Polya's Problem Solving Techniques and Duke’s 7 steps. In particular, he/she will be able to
- describe the role of abstraction in analyzing a problem description,
- describe the difference between clarifying and probing questions, and
- understand the importance of basic data structure and algorithm knowledge.
- Apply problem-solving strategies to coding interview problems, including: abstraction, question generation (clarifying and probing), data collection and analysis, problem decomposition, and pattern generalization.
- Communicate (oral and written) solutions to technical/coding problems.
- Solve technical/coding problems with redundant, incomplete, and inconsistent specifications.
- Evaluate correctness and quality of different solutions to technical/coding problems using metrics such as efficiency, correctness, and coverage.
- Work efficiently in groups to provide solutions to problems, defend decisions and accept/provide constructive critique of solutions presented by other groups.
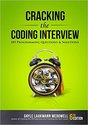
- Text Book:
- McDowell, Gayle Laakmann. Cracking the Coding Interview: 189 Programming Questions and Solutions. CareerCup, LLC, 2016. http://www.crackingthecodinginterview.com
- Books:
- Mongan, John, Noah Suojanen Kindler, and Eric Giguère. Programming interviews exposed: secrets to landing your next job. John Wiley & Sons, 2012.
- Robert Sedgewick. Algorithms in C++, Third Edition. Addison Wesley. 1998.
- T.H. Cormen, C.E. Leiserson, R. L. Rivest, and C. Stein Introduction to Algorithms (2nd Edition), The MIT Press, Mc-Graw-Hill (2001)
- http://www.cplusplus.com/doc/language/tutorial/structures.html
- A.V. Aho, J.E. Hopcroft, and J.D. Ullman: Data Structures and Algorithms, Addison-Wesley (1983)
- Donald E. Knuth: The Art of Computer Programming, Addison-Wesley (1997)
- Links:
- I. Koutis, Fun with Programming Interview Questions - Course Syllabus http://ccom.uprrp.edu/~ikoutis/classes/fun_15/Fun_15.htm
- Project Euler. https://projecteuler.net
- LeetCode. https://leetcode.com
- Pat Morin. Open Data Structures. Licensed under a Creative Commons License. Available at: http://www.aupress.ca/books/120226/ebook/03_Morin_2013-Open_Data_Structures.pdf
- Programming Abstractions (Stanford University) - http://www.stanford.edu/class/cs106b/
- Notes for the Sedgewick's Algorithms book: http://algs4.cs.princeton.edu/home/